Make your browser read to you using the web speech API
Table of contents
In the last decades, browsers have been developed to become the Joker application used for everything. Especially with the advent of HTML 5, a lot of APIs became available on the web.
Some of those APIs are made to help developers to build various voice applications and improve user experience in browsers.
In this article, we will see how you can take advantage of the Web Speech API by building your own text reader using JavaScript.
So, what is the Web Speech API?
Well In general, browser APIs are interfaces and predefined functionalities built within the browser in order to provide native features and extensions for web applications. They give the developer a set of tools and methodologies to use while building fast, scalable, and efficient web applications.
Among those APIs, we have the Web Speech API which enables you to incorporate voice data into your web applications. This API has two interfaces:
- SpeechSynthesis: for text-to-speech, it gives us the ability to read text content using the speech synthesizer in the target device.
- SpeechRecognition: for speech input and recognition, it allows us to recognize the voice from an audio input.
The easy way
Lot of technical words I know, but no worries, in this section, I will give you the minimal JS code you need for your browser to speak:
let speaker = new SpeechSynthesisUtterance("Hi there, I am speaking !");
window.speechSynthesis.speak(speaker);
If you are in a hurry, just paste those lines into your console. It will work just fine. Otherwise, you can keep reading to get a detailed explanation.
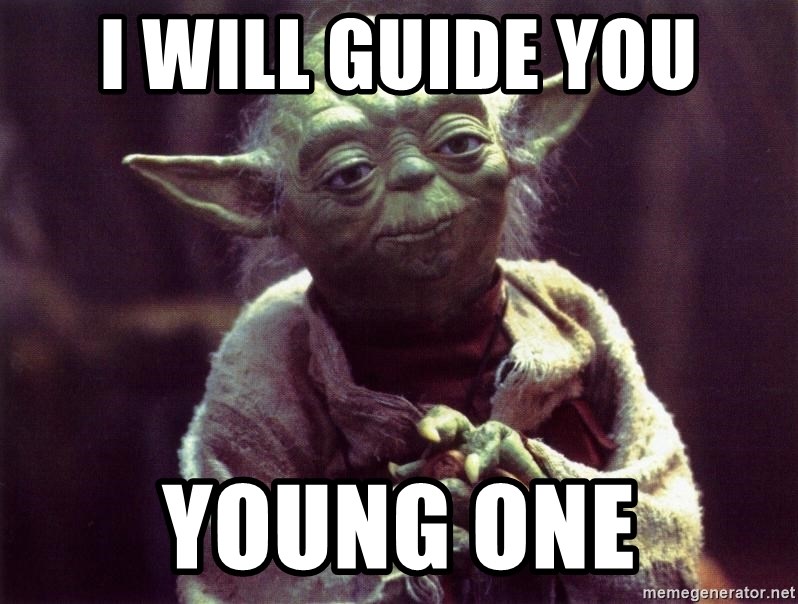
In the first line, we instantiated the SpeechSynthesisUtterance interface. It contains the content the speech service should read and information about how to read it such as language, voice, pitch, and rate ...
In the next line we use the speak method inside the speechSynthesis interface, to start reading the text with the given utterance params.
Let’s make it interactive
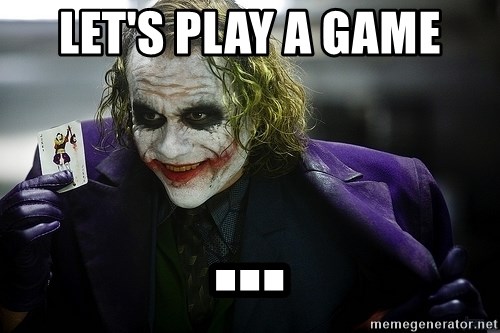
Now let’s make our game interactive by taking input from the user. First of all we need an input or a textarea field to get input from the user:
<textarea id="content"></textarea>
<button id="play-btn">Play</button>
Now we will target our elements using JS. The scenario is when the play button is pressed, the content of the textarea will be read.
const textarea = document.getElementById("content");
const playBtn = document.getElementById("play-btn");
const speaker = new SpeechSynthesisUtterance();
playBtn.addEventListener("click", () => {
readText(textarea.value);
});
After targeting all needed elements, we will define the readText function:
function readText(text) {
speaker.text = text;
speaker.volume = 1; // From 0 to 1
speaker.rate = 1; // From 0.1 to 10
speaker.lang = "en";
speechSynthesis.speak(speaker);
}
You can also let the user set utterance options like volume, rate, lang, voice ... using other input fields. And here is a great example from the “web dev simplified” youtube channel.
Browser voice applications
In this chapter I want to give you a list of some cool projects you may love to try using the Web Speech API:
- Quotes reader: In this app, you may want to get a random quote from an API and read it using the speechSynthesis interface.
- Voice assistant: You wanna build your own Alexa version in the browser, which takes a simple voice predefined list of orders and tries to recognize what to do using the SpeechRecognition interface.
Conclusion
In this article, we’ve covered how to use the Web Speech API in order to create voice-based web applications in the browser.
We started with a simple working code snippet, then we added some kind of interactivity by taking input from users.
In the end, we suggested some ideas you may be interested in working on using the Web Speech API.
I hope that everything is clear. Now let me hear your browser. If you have any questions, remarks or additional information, feel free to contact me.
Before I quit, I just wanted to thank my friend @Tahabsn for his time, help, and guidance.
Have a nice day ^_^